What does the following code do? The first code listing contains the declaration of the Shape class. Each Shape object represents a closed shape with a number of sides. The second code listing contains a method (Mystery) created by a client of the Shape class. What does this method do?
What will be an ideal response?
// Shapes.cs
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace Shapes
{
///
/// Summary description for FrmShapes.
///
public class FrmShapes : System.Windows.Forms.Form
{
private System.Windows.Forms.Label lblFiveSidesOut;
private System.Windows.Forms.Label lblFourSidesOut;
private System.Windows.Forms.Label lblThreeSidesOut;
private System.Windows.Forms.Label lblTwoSidesOut;
private System.Windows.Forms.Label lblFiveSides;
private System.Windows.Forms.Label lblFourSides;
private System.Windows.Forms.Label lblThreeSides;
private System.Windows.Forms.Label lblTwoSides;
///
/// Required designer variable.
///
private System.ComponentModel.Container components = null;
public FrmShapes()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent
// call
//
}
///
/// Clean up any resources being used.
///
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
// Windows Form Designer generated code
///
/// The main entry point for the application.
///
[STAThread]
static void Main()
{
Application.Run( new FrmShapes() );
}
// calls Mystery to and prints the results
private void FrmShapes_Load(
object sender, System.EventArgs e )
{
Shape objLine = new Shape( 2 );
Shape objTriangle = new Shape( 3 );
Shape objSquare = new Shape( 4 );
Shape objPoly = new Shape( 5 );
lblTwoSidesOut.Text = Mystery( objLine );
lblThreeSidesOut.Text = Mystery( objTriangle );
lblFourSidesOut.Text = Mystery( objSquare );
lblFiveSidesOut.Text = Mystery( objPoly );
} // end method FrmShapes_Load
// returns the name of a shape based on its number of sides
public string Mystery( Shape objShape )
{
string strShape;
switch ( objShape.Side )
{
case 0:
case 1:
case 2: strShape = "Not a Shape";
break;
case 3: strShape = "Triangle";
break;
case 4: strShape = "Square";
break;
default: strShape = "Polygon";
break;
}
return strShape;
} // end method Mystery
} // end class FrmShapes
}
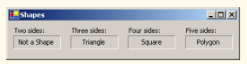
Computer Science & Information Technology
You might also like to view...
To go to a particular Web site on the Internet you must type the ________ in the Internet address bar.
a. URL (Universal Resource Locator) b. connection locator c. site name d. routeB
Computer Science & Information Technology
For security purposes you want to have data redundancy in your tables
Indicate whether the statement is true or false
Computer Science & Information Technology