The following code gives an example of a C program that calls a function.
void adder(int a, int *b)
{
*b = a + *b;
}
void main (void)
{
int x = 3, y = 4;
adder(x, &y);
}
This 68000 program creates the following output from a compiler. The panel describes some of the instructions that are not obvious.
*1 void adder(int a, int *b)
Parameter a is at 8(FP)
Parameter b is at 10(FP)
adder
LINK FP,#0
*2 {
*3 *b = a + *b;
MOVEA.L 10(FP),A4
MOVE (A4),D1
ADD 8(FP),D1
MOVE D1,(A4)
*4 }
UNLK FP
RTS
*5
*6 void main (void)
Variable x is at -2(FP)
Variable y is at -4(FP)
main
LINK FP,#-4
*7 {
*8 int x = 3, y = 4;
MOVE #3,-2(FP)
MOVE #4,-4(FP)
*9 adder(x, &y);
PEA -4(FP)
MOVE #3,-(A7)
JSR adder
*10
*11 }
UNLK FP
RTS
Draw the state of the stack immediately after function adder is called in function main (i.e., the return address is on the top of the stack but no code in adder has yet been executed). Carefully label all items on the stack and give their location with respect to the current value of the frame pointer.
Draw a sequence of diagrams (i.e., memory maps) showing what happens to the stack as function adder is executed. Explain the action of each instruction that modifies the state of the stack. Show how parameters are passed to and from the function and how stack?based values are accessed.
The code of main is:
Main LINK FP,#-4
MOVE #3,-2(FP)
MOVE #4,-4(FP)
PEA -4(FP)
MOVE #3,-(A7)
JSR adder
UNLK FP
RTS
The following diagram gives the state of the stack after the subroutine call (the leftmost picture)
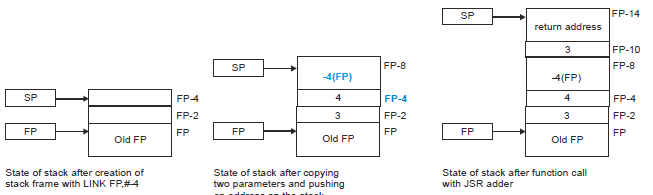
The code of the function adder is below. A dummy frame pointer is first created (i.e., no new storage is allocated but the frame pointer points to the base of this frame.
Adder LINK FP,#0
MOVEA.L 10(FP),A4
MOVE (A4),D1
ADD 8(FP),D1
MOVE D1,(A4)
UNLK FP
RTS
The figure below gives the state of the stack after the creation of a stack frame in adder. Note the new numbering of addresses with respect to the current frame pointer. Now you can see how the code works. For example, MOVEA.L 10(FP),A4 loads the 4?byte contents of memory 10 bytes below the FP into A4. This value is the address of the 4 pushed on the stack in location FP+14. So, when MOVE (A4),D4 is executed, the value 4 is loaded into D1.
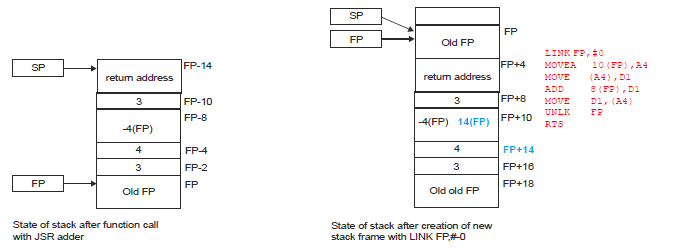
Computer Science & Information Technology
You might also like to view...
Insights pane is a document view in Microsoft Word that distinguishes the importance of data by the heading styles that are applied
Indicate whether the statement is true or false
Computer Science & Information Technology
A file name extension indicates the file format.
Answer the following statement true (T) or false (F)
Computer Science & Information Technology