Find the error(s) in the following code. The event handler should have an agent object appear and say, “Hello, my name is Merlin.” This should happen when the user clicks the Call Button.
private void btnCall_Click( object sender, System.EventArgs e )
{
AgentObjects.IAgentCtlCharacter objMSpeaker;
objMainAgent.Characters.Load( "Merlin", "Merlin.acs" );
objMSpeaker = objMainAgent.Characters[ "Merlin.acs" ];
objMSpeaker.Show( 0 );
objMSpeaker.Play( "Hello, my name is Merlin", "" );
} // end method btnCall_Click
To have the agent say “Hello, my name is Merlin”, you must call the Speak method
rather than the Play method. Also, the name of the character should be passed on line 7, not the name of the file that defines the character. The complete incorrect code reads:
// Merlin.cs (Incorrect)
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace Merlin
{
///
/// Summary description for FrmMerlin.
///
public class FrmMerlin : System.Windows.Forms.Form
{
private System.Windows.Forms.Label lblCall;
private System.Windows.Forms.Button btnCall;
private AxAgentObjects.AxAgent objMainAgent;
///
/// Required designer variable.
///
private System.ComponentModel.Container components = null;
public FrmMerlin()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent
// call
//
}
///
/// Clean up any resources being used.
///
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
// Windows Form Designer generated code
///
/// The main entry point for the application.
///
[STAThread]
static void Main()
{
Application.Run(new FrmMerlin());
}
// calls "Merlin" the agent
private void btnCall_Click( object sender, System.EventArgs e )
{
AgentObjects.IAgentCtlCharacter objMSpeaker;
objMainAgent.Characters.Load( "Merlin", "Merlin.acs" );
objMSpeaker = objMainAgent.Characters[ "Merlin.acs" ];
objMSpeaker.Show( 0 );
objMSpeaker.Play( "Hello, my name is Merlin", "" );
} // end method btnCall_Click
} // end class FrmMerlin
}
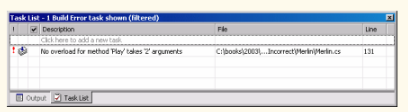
The complete corrected code should read:
// Merlin.cs (Correct)
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace Merlin
{
///
/// Summary description for FrmMerlin.
///
public class FrmMerlin : System.Windows.Forms.Form
{
private System.Windows.Forms.Label lblCall;
private System.Windows.Forms.Button btnCall;
private AxAgentObjects.AxAgent objMainAgent;
///
/// Required designer variable.
///
private System.ComponentModel.Container components = null;
public FrmMerlin()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent
// call
//
}
///
/// Clean up any resources being used.
///
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
// Windows Form Designer generated code
///
/// The main entry point for the application.
///
[STAThread]
static void Main()
{
Application.Run( new FrmMerlin() );
}
// calls "Merlin" the agent
private void btnCall_Click( object sender, System.EventArgs e )
{
AgentObjects.IAgentCtlCharacter objMSpeaker;
objMainAgent.Characters.Load( "Merlin", "Merlin.acs" );
objMSpeaker = objMainAgent.Characters[ "Merlin" ];
objMSpeaker.Show( 0 );
objMSpeaker.Speak( "Hello, my name is Merlin", "" );
} // end method btnCall_Click
} // end class FrmMerlin
}

Computer Science & Information Technology
You might also like to view...
A(n) _________ specific item that can be cataloged in Active Directory
a. Object b. Class c. Attribute d. None of the above.
Computer Science & Information Technology
When using ____, you can develop new classes more quickly by extending existing classes that already work.
A. inheritance B. polymorphism C. encapsulation D. construction
Computer Science & Information Technology