This method should assign a random decimal number (in the range 0 to Int32.Max- Value) to decimal decNumber. Find the error(s) in the following code.
void RandomDecimal()
{
decimal decNumber;
Random objRandom = new Random();
decNumber = objRandom.NextDouble();
lblDisplay.Text = Convert.ToString( decNumber );
} // end method RandomDecimal
Random objects can produce ints and doubles only; this will yield only an int ran-
dom number. [Note: There is no way to have the Random class generate a decimal random value, so the solution is a bit contrived. The closest approximation is to use NextDouble.]
The result also must be multiplied by Int32.MaxValue to achieve the correct range of values. The complete incorrect code reads:
// RandomNumber.cs (Incorrect)
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace RandomNumber
{
///
/// Summary description for FrmRandomNumber.
///
public class FrmRandomNumber : System.Windows.Forms.Form
{
private System.Windows.Forms.Label lblDisplay;
private System.Windows.Forms.Label lblRandom;
///
/// Required designer variable.
// RandomNumber.cs (Incorrect)
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace RandomNumber
{
///
/// Summary description for FrmRandomNumber.
///
public class FrmRandomNumber : System.Windows.Forms.Form
{
private System.Windows.Forms.Label lblDisplay;
private System.Windows.Forms.Label lblRandom;
///
/// Required designer variable.
decNumber = objRandom.NextDouble();
lblDisplay.Text = Convert.ToString( decNumber );
} // end method RandomDecimal
} // end class FrmRandomNumber
}
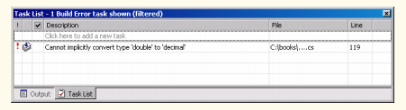
The complete corrected code should read:
// RandomNumber.cs (Correct)
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace RandomNumber
{
///
/// Summary description for FrmRandomNumber.
///
public class FrmRandomNumber : System.Windows.Forms.Form
{
private System.Windows.Forms.Label lblDisplay;
private System.Windows.Forms.Label lblRandom;
///
/// Required designer variable.
///
private System.ComponentModel.Container components = null;
public FrmRandomNumber()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent
// call
//
}
///
/// Clean up any resources being used.
///
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
// Windows Form Designer generated code
///
/// The main entry point for the application.
///
[STAThread]
static void Main()
{
Application.Run( new FrmRandomNumber() );
}
// calls the RandomDecimal helper method
private void FrmRandomNumber_Load(
object sender, System.EventArgs e )
{
RandomDecimal();
} // end method FrmRandomNumber_Load
// generates a random decimal which it then displays
void RandomDecimal()
{
decimal decNumber;
Random objRandom = new Random();
decNumber = ( Int32.MaxValue *
Convert.ToDecimal( objRandom.NextDouble() ) );
lblDisplay.Text = Convert.ToString( decNumber );
} // end method RandomDecimal
} // end class FrmRandomNumber
}
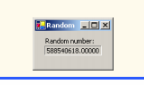
Computer Science & Information Technology