The following code should add integers from two TextBoxes and display the result in txtResult. Assume that intValue1 and intValue2 are declared as ints. Find the error(s) in the following code:
try
{
intValue1 = Int32.Parse( txtInput1.Text );
intValue2 = Int32.Parse( txtInput2.Text );
txtOutput.Text = Convert.ToString( intValue1 + intValue2 );
catch ( )
{
MessageBox.Show(
"Please enter valid ints.",
"Invalid Number Format",
MessageBoxButtons.OK, MessageBoxIcon.Error );
}
}
There are two errors in this code. First, the catch block appears inside the try
block. All catch blocks must follow immediately after the try block. Second, you will need to specify which type of exception is being caught between the parentheses after the catch statement (in this case, a FormatException). The complete incorrect code reads:
// Addition.cs (Incorrect)
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace Addition
{
///
/// Summary description for FrmAddition.
///
public class FrmAddition : System.Windows.Forms.Form
{
// Label and TextBox for first value
private System.Windows.Forms.Label lblValue1;
private System.Windows.Forms.TextBox txtInput1;
// Label and TextBox for second value
private System.Windows.Forms.Label lblValue2;
private System.Windows.Forms.TextBox txtInput2;
// Label and TextBox for output
private System.Windows.Forms.Label lblProduct;
private System.Windows.Forms.TextBox txtOutput;
// Button to perform addition
private System.Windows.Forms.Button btnAdd;
///
/// Required designer variable.
///
private System.ComponentModel.Container components = null;
public FrmAddition()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent
// call
//
}
///
/// Clean up any resources being used.
///
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
// Windows Form Designer generated code
///
/// The main entry point for the application.
///
[STAThread]
static void Main()
{
Application.Run( new FrmAddition() );
}
// handles btnAdd's Click event
private void btnAdd_Click(
object sender, System.EventArgs e )
{
int intValue1;
int intValue2;
try
{
intValue1 = Convert.ToInt32( txtInput1.Text );
intValue2 = Convert.ToInt32( txtInput2.Text );
txtOutput.Text =
Convert.ToString( intValue1 + intValue2 );
catch ( )
{
MessageBox.Show(
"Please enter valid integers.",
"Invalid Number Format",
MessageBoxButtons.OK, MessageBoxIcon.Error );
}
}
} // end method btnAdd_Click
} // end class FrmAddition
}
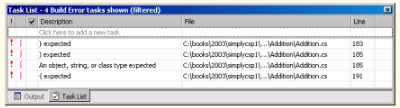
The complete corrected code should read:
// Addition.cs (Correct)
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace Addition
{
///
/// Summary description for FrmAddition.
///
public class FrmAddition : System.Windows.Forms.Form
{
// Label and TextBox for first value
private System.Windows.Forms.Label lblValue1;
private System.Windows.Forms.TextBox txtInput1;
// Label and TextBox for second value
private System.Windows.Forms.Label lblValue2;
private System.Windows.Forms.TextBox txtInput2;
// Label and TextBox for output
private System.Windows.Forms.Label lblProduct;
private System.Windows.Forms.TextBox txtOutput;
// Button to perform addition
private System.Windows.Forms.Button btnAdd;
///
/// Required designer variable.
///
private System.ComponentModel.Container components = null;
public FrmAddition()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent
// call
//
}
///
/// Clean up any resources being used.
///
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
// Windows Form Designer generated code
///
/// The main entry point for the application.
///
[STAThread]
static void Main()
{
Application.Run( new FrmAddition() );
}
// handles btnAdd's Click event
private void btnAdd_Click(
object sender, System.EventArgs e )
{
int intValue1;
int intValue2;
try
{
intValue1 = Int32.Parse( txtInput1.Text );
intValue2 = Int32.Parse( txtInput2.Text );
txtOutput.Text =
Convert.ToString( intValue1 + intValue2 );
}
catch ( FormatException formatExceptionParameter )
{
MessageBox.Show(
"Please enter valid integers.",
"Invalid Number Format",
MessageBoxButtons.OK, MessageBoxIcon.Error );
}
} // end method btnAdd_Click
} // end class FrmAddition
}
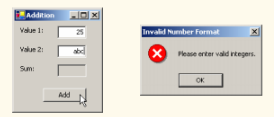
You might also like to view...
When entering the InputBox function in the Code Editor window, the prompt, title, and defaultResponse arguments must be enclosed in ____.
A. quotation marks B. asterisks C. square brackets D. slashes
If you click the Select button in the accompanying figure, which of the following happens?
A. Dreamweaver connects to the remote server. B. The names of all the databases on the remote server to which you have access appear in the Select Database list. C. The Select Database dialog box opens. D. All of the above